In the rapidly evolving world of AI and machine learning, the ability to personalize and extend the capabilities of models like ChatGPT is becoming increasingly important. One exciting development in this area is the introduction of a feature that allows ChatGPT to utilize a customized knowledge base. This presents an opportunity to tailor the model’s responses more closely to specific domains or use cases.
To facilitate this, we need a way to efficiently convert a repository of Python code into a format that can be ingested by the model. The following is a practical guide on how to merge Python files from a directory into text files, ready to be used as part of a customized knowledge base for ChatGPT.
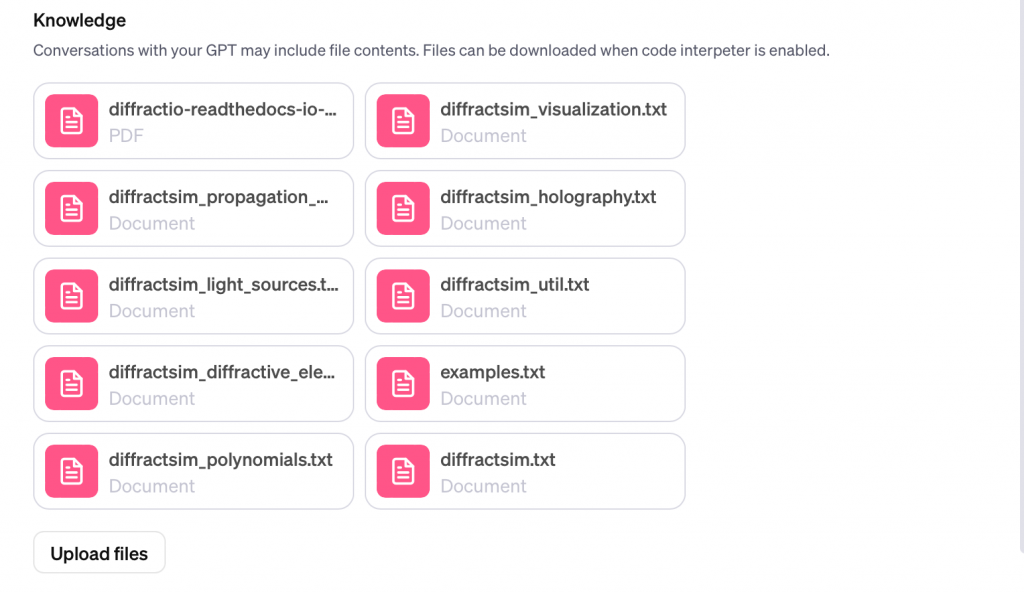
Table of Contents
The Context
Imagine you have a repository filled with Python scripts, each containing valuable snippets of code and information. You want to leverage this repository to enhance ChatGPT’s understanding in a particular domain. The challenge lies in transforming this codebase into a format that is compatible with ChatGPT’s new feature.
The Solution
The code snippet provided here is a simple yet effective Python script designed to merge multiple Python files from a specified directory into single text files. This conversion process is crucial for preparing the codebase for integration with ChatGPT’s knowledge base.
How It Works
- Walking Through Directories: The script uses
os.walk
to navigate through the specified source directory, identifying Python files (.py
extensions). - Merging Files: It then combines all Python files found in each subdirectory into a single text file. The path of each original file is included for easy reference.
- Organizing Output: The merged files are named based on their relative paths in the source directory, ensuring a structured and understandable output.
- Flexibility: The script is adaptable – you can specify any source and target directory, making it versatile for different projects.
The Code
import os
def merge_py_files_by_directory(source_directory, target_directory):
for subdir, dirs, files in os.walk(source_directory):
py_files = [f for f in files if f.endswith('.py')]
if py_files:
relative_path = os.path.relpath(subdir, start=source_directory)
new_filename = relative_path.replace(os.sep, '_') + '.txt'
target_file_path = os.path.join(target_directory, new_filename)
os.makedirs(target_directory, exist_ok=True)
with open(target_file_path, "w") as outfile:
for file in py_files:
file_path = os.path.join(subdir, file)
outfile.write(f"{'=' * 20}\n")
outfile.write(f"File: {file_path}\n")
outfile.write(f"{'=' * 20}\n\n")
with open(file_path, "r") as infile:
outfile.write(infile.read())
outfile.write("\n\n")
# Example usage
source_directory = 'diffraction'
target_directory = 'merged_py_files'
merge_py_files_by_directory(source_directory, target_directory)
Conclusion
This approach offers a streamlined and effective method for converting a Python code repository into a format suitable for enhancing ChatGPT’s custom knowledge base. By following this guide, you can harness the power of your existing codebase to create a more informed and domain-specific AI model.